JQuery Tutorial #5: Search HTML Elements with jQuery Selectors
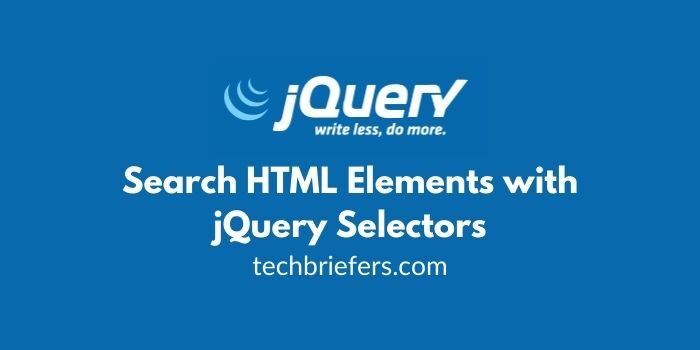
After getting to know the Rules Of Writing JQuery Code in the previous tutorial, this time I will discuss how to find HTML elements with jQuery Selector.
Understanding JavaScript’s getElementById() function
As is the main function of JavaScript, we need JavaScript to manipulate HTML elements. The first step is to find which element to change, whether it is a paragraph tag, that is <p> or a <p> tag with the id=”important” attribute, a <p> tag with the class=”warning” attribute , or a <button> button .
In JavaScript, to find HTML elements, we usually use the getElementById()
method. This method or function is used to find HTML elements based on their id attribute.
For example, if I have the following HTML code:
<button id = "button"> Click Me! </button>
So to access this element we can use:
var x = document.getElementById ("button");
By using the jQuery Selector , the program code is much shorter and more powerful.
Understanding jQuery Selector
jQuery simplifies the search process for HTML elements by providing a jQuery Selector . The jQuery Selector is a way that jQuery provides to search HTML elements. Not only with the id attribute, we can also use classes, other attributes, and a combination thereof.
If you have used CSS before, jQuery borrows how to write CSS Selector as jQuery Selector.
With jQuery, to find an HTML element that has id="button”
, the writing is much shorter:
var x = $("#button");
Because just like CSS selectors, jQuery selector supports almost all writing selectors from CSS, here is an example:
- Search for all elements with a specific tag. Suppose we want to find all <p> tags, writing the jQuery Selector is: $(“p”).
- Search for elements with a specific id. Suppose we want to find an HTML tag that has id=”study”, then we can write: $(“#study”).
- Search for elements with a certain class. Suppose we want to find all HTML tags that use the class=”color” attribute, then it can be written as: $(“.color”).
Just like CSS, we can also combine selectors to find a more specific element, for example, to find a <p> tag that has class=”color” and is inside the <div> tag , the selector is: $(“div p.color”) .
Here’s an example of using the jQuery Selector:
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>jQuery Tutorial | Techbriefers</title>
<script src="jquery-3.6.0.min.js"></script>
</head>
<body>
<p>
A paragraph without any attributes
</p>
<p id="study">
This paragraph has attribute id = "study"
</p>
<p class="color">
This paragraph has attribute class = "color"
</p>
<p id="test" class="only">
This paragraph has two attributes id="test" class="only"
</p>
<div>
<p class="color">
This paragraph is inside the div with attribute class=”color”
</p>
</div>
<button id="button">Click for Action</button>
<script>
$(document).ready(function () {
$("#button").click(function () {
$("p").css("color", "red");
$("#study").css("color", "green");
$(".color").css("color", "blue");
$("#test.only").css("color", "yellow");
$("div p.color").css("color","orange");
});
});
</script>
</body>
</html>
The code output will like the image below, in the browser.
After Running the jQuery, that is by Clicking on the action button, The outcome will be as in the following image.
Explanation of the example of jQuery Selectors
In the sample code above, there are 6 writing jQuery Selectors. Can you find it? The six selectors are:
$("#button")
$("p")
$("#study")
$(".color")
$("#test.only")
$("div p.color")
The first selector, $(“# button”) I used, to create a click event. That is, when an HTML tag that has id=”button” is clicked, run the command that is created. In the code above, the HTML tag is a <button id=”button”> tag.
We will discuss more about the click() event/function in the next tutorial. This time we only focus on how to write the jQuery Selectors.
After clicking the <button id = “button”> tag, I created 5 lines of command, each of which is used to change the color of the paragraph:
$("p").css( "color", "red" );
$("#study").css( "color", "green" );
$(".color").css( "color", "blue" );
$("#test.only").css( "color", "yellow" );
$("div p.color").css( "color", "orange" );
For example, the code $ (“#study”).css(“color”, “green”) means: find an HTML tag with id=”study”, then change its color (color) to green (green). Again, here we only focus on how to write selectors. The css() event will be studied in a separate tutorial.
In these 5 lines, I changed the color of each paragraph, of course, with different selectors.
Please test via the following codepen link:
See the Pen yLgdNEq by Techbriefers (@techbriefers) on CodePen.
The jQuery Selectors that we learn are very important to understand because this is where we look for which elements we want to manipulate with jQuery. Next, I will go into the jQuery Event, which is what we can do with an HTML element.
Leave a Reply