JQuery Tutorial # 6: Understanding jQuery Events and How to Use them
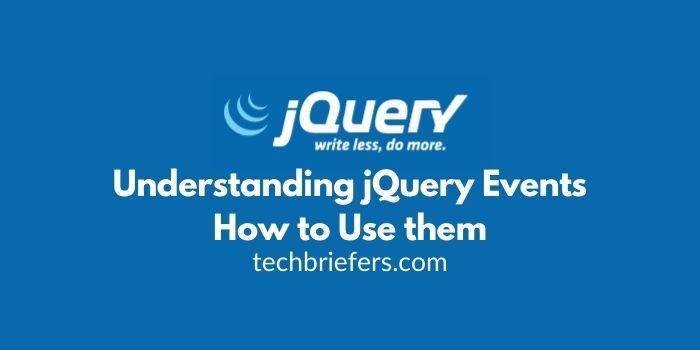
JQuery Selector and jQuery Event are at the heart of jQuery. By combining the two, we can make a variety of interesting interactions. In this jQuery learning tutorial, I will discuss further the Understanding and How to Use the jQuery Event.
Understanding jQuery Event
In JavaScript, an event is something that a user can do to an HTML element, for example clicking , double clicking , mouseover (the mouse cursor is above the element), mouseout (the mouse cursor has left the element), etc.
Suppose I want to create an alert() message when a paragraph is clicked, with JavaScript this can be done with the following program code:
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>JQuery Tutorial | Techbriefers</title>
</head>
<body>
<p id="mypara" onclick="alert(You clicked this paragraph!')">
I am a paragraph, Click me.
</p>
</body>
</html>
The onclick attribute in the <p> tag above is an Event. When this paragraph will be clicked, a popup message will appear: ‘I’ve been clicked!’. These results are obtained with the JavaScript alert() function.
Apart from onclick, in JavaScript, there are also many other events, such as ondblclick, onmouseover, onmouseout , etc.
Writing like this is very practical but not “neat”. Because we mix HTML code with JavaScript. How to separate them?
Inside JavaScript, this can be done using the addEventListener () function . The same result can be produced with the following program code:
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>JQuery Tutorial | Techbriefers</title>
<script src="jquery-3.6.0.js"></script>
<script>
window.onload = function()
{
document.getElementById("mypara").
addEventListener("click", function(){alert ('You clicked this paragraph!')});
}
</script>
</head>
<body>
<p id="mypara">
I am a paragraph, Click me.
</p>
</body>
</html>
Now, inside the <body> section there isn’t any JavaScript code. I added the click event with the addEventListener() function. Regarding the window.onload() function, I have discussed it in the JavaScript Tutorial: Best Position to Put JavaScipt code in HTML.
How to Use the jQuery Event
As an alternative, jQuery provides a jQuery Event with a more practical writing, here is an example:
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>JQuery Tutorial | Techbriefers</title>
<script src="jquery-3.6.0.js"></script>
<script>
$(document).ready(function() {
$("#mypara").click(function() {
alert('You clicked this paragraph!');
});
});
</script>
</head>
<body>
<p id="mypara">
I am a paragraph, Click me.
</p>
</body>
</html>
Notice how jQuery makes it easy to write this event, it’s really simple. The writing format is:
$(“jQuery_Selector”).jQuery_Event(function() { …write your event here… }) |
Apart from the click event, there are also various other events available, such as dblclick , mouseenter , mouseover , mouseleave , etc.
Complete Example for Understanding jQuery Events
Here’s an example of the “action” of various JavaScript events created with jQuery:
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>JQuery Tutorial | Techbriefers</title>
<style>
#mypara {
width: 600px;
height: 100px;
background-color: pink;
line-height: 100px;
text-align: center;
font-size: 30px;
margin: auto;
}
</style>
<script src="jquery-3.6.0.js"></script>
<script>
$(document).ready(function() {
$("#mypara").click(function() {
$("#mypara").css( "color", "green" );
});
$("#mypara").mouseover(function() {
$("#mypara").css( "background-color", "silver" );
});
$("#mypara").mouseout(function() {
$("#mypara").css( "background-color", "pink" );
});
$("#mypara").dblclick(function() {
$("#mypara").css( "border", "solid 2px black" );
});
});
</script>
</head>
<body>
<p id="mypara">
Understanding jQuery Events
</p>
</body>
</html>
In the example above, I created 4 jQuery events, namely click, dblclick, mouseover, and mouseleave. In each of these events I change the CSS property of the paragraph. Please try it yourself by clicking, double clicking, and mouseover over the pink box:
See the Pen Understanding jQuery Events and How to Use them by Techbriefers (@techbriefers) on CodePen.
Throughout this jQuery tutorial, we will discuss many things related to jQuery Events, including animated events such as show(), hide(), etc.