Jquery Tutorial #3: How to Run jQuery Code (event ready)
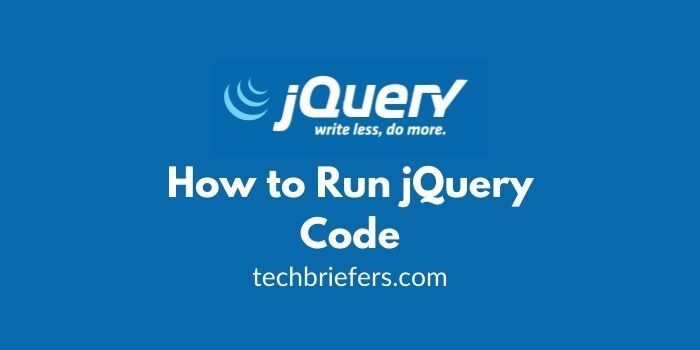
In a previous tutorial, we discussed How To Link JQuery Files Into HTML, either by downloading a jQuery file (to run offline) or from a CDN.
This time I will continue by discussing how to run jQuery code. JQuery code is generally placed inside the ready () event.
How to run jQuery Code
As we discussed, jQuery is actually JavaScript. Thus, the code that is written is JavaScript code. JQuery only ‘makes it easy’ to write JavaScript with a variety of built-in functions.
To input JavaScript code with a jQuery ‘flavor’, the most common way is to place it after the <script> tag which is used to call the jQuery file, as in the following example:
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>How to Run Jquery COde</title>
<script src="jquery-2.1.4.js"></script>
<script>
// JavaScript and jQuery code here
</script>
</head>
<body>
<!-- HTML Code here -->
</body>
</html>
With the ‘template’ above, we can actually run jQuery functions right away. However, there is 1 function that is always included in every jQuery code, namely the ready () event .
Definition of Event ready or The Document Ready Event
To understand jQuery’s ready () function or formally referred to as The Document Ready Event, we must understand how JavaScript works.
In simple terms, JavaScript is used to manipulate HTML objects such as paragraphs, buttons, forms, images, etc. If we place the JavaScript code at the beginning (in the <head> section), chances are that this HTML object is not yet available. This happens because the <body> section is only processed after the <head> section.
One solution to this is to put the JavaScript code in the closing section of the page, that is, before the closing </body> tag. However, jQuery has a more elegant solution, namely event ready().
If you don’t understand this explanation, you can read our JavaScript tutorial: Best Position to Put JavaScript code in HTML.
Demo of how to Run jQuery Code
The jQuery ready () event simulates the effect of the JavaScript Onload event, but is more efficient. By using jQuery’s ready() function, the new JavaScript code will be executed after the web browser has finished processing the HTML tag, but before any external files such as images. Thus, JavaScript code is processed earlier than JavaScript’s built-in Onload event. The writing of jQuery’s ready () function is as follows:
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Learn JQuery</title>
<script src="jquery-3.6.0.min.js"></script>
<script>
$(document).ready(function() {
// JQuery code Here
});
</script>
</head>
<body>
</body>
</html>
The JavaScript code that is inside the $ (document) .ready (function ()) function will be executed after all HTML tags have been processed by the web browser. Thus, we can avoid errors that occur because the object we want to manipulate is not available.
If you look at the tutorials on jQuery, it’s almost certain that all of the code will be inside this ready () event.
For example, I will create a simple program code, where when the HTML button is clicked, a sentence will appear. Here’s the program code:
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Learn JQuery</title>
<script src="jquery-3.6.0.min.js"></script>
<script>
$(document).ready(function() {
$("#button").click(function() {
$(this).after('<p> How to Run jQuery Code </p>');
});
});
</script>
</head>
<body>
<h1> How to Run jQuery Code </h1>
<button id="button">Click Me!</button>
</body>
</html>
I will discuss the purpose of the code above in the next tutorial. Here we only focus so you can run jQuery code.
In order to run, save the above program code in the same folder as the jquery-3.6.0.min.js file. For example, I will save them into a file jqt3.html in folders jquery , such as the following:
Here is the final result of the code above (please click the Click Me button!):
Leave a Reply