How to work with Session and Cookies in CodeIgniter
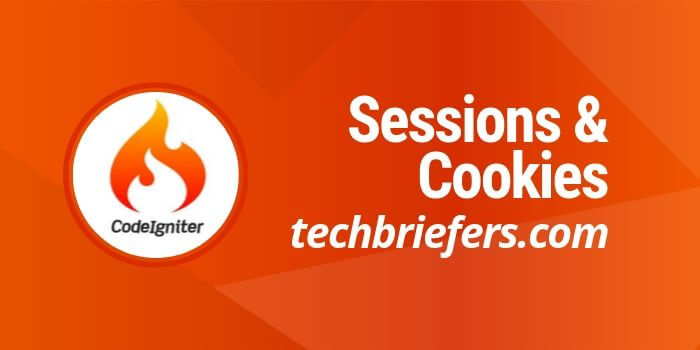
Cookies and sessions are the two major concepts for PHP or any CodeIgniter web framework. So today I will discuss about cookies as well as a session in CodeIgniter, in this tutorial. This article has a complete focus on understanding the concept of cookies and sessions. So, first of all, let’s start with the diagram which is present as the pictorial representation of how cookies and sessions are managed.
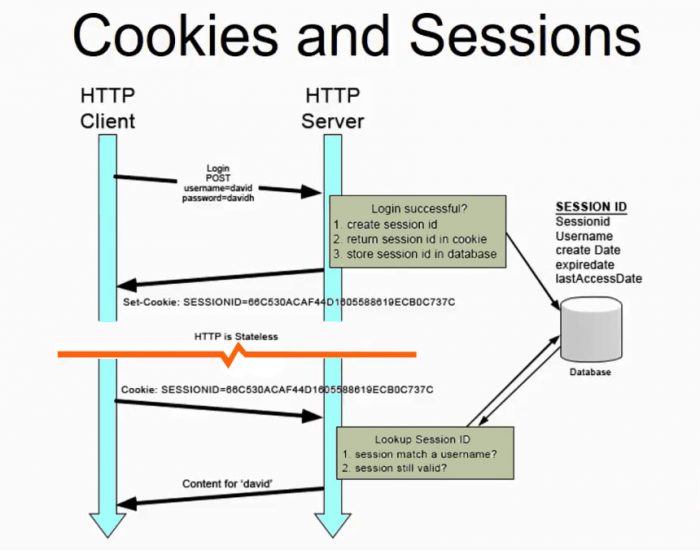
It is like we have the client and server application structure and cookies are the ones who interact from the client perspective. It includes all the user information in it which has the information related to this session activity as well. So both are interdependent also somewhere like and connected to each other when it comes to the transformation law or you can say the communication from the client-server end. So we have the HTTP client over here as you can see in the diagram.
All the cookies, as well as the sessions, are completely dependent on this client-server communication like the two-way client-server communication or the one-way or whatever the requested responds us in it is all managed with the help of cookies and sessions. Now the basic concept comes in our mind is how cookies and sessions interact with each other. And how they are managed? We have this picture above, and you see that there is an HTTP client.
You can see the client is nothing but the one which manages the user information. It saves the user information and it waits for the RIC responds and sends information to this server. For example login, I’ve given an example over here with a username and password. It will send this request to the HTTP server to check whether my login and password are correct or not. Now, what the HTTP server does is: there are two database servers. It will check the session ID, the username; create date, expiry date, last access date and all various parameters to the database. We can say, the session is nothing but the particular stipulated time period where all the users interact with each other.
Cookies and sessions concept in CodeIgniter
Cookies and sessions are based on the perspective that cookies include the information which is through the client to server, which is nothing but the request information. The request is then later verified, not under these servers for the information that you provide, to be correct or not. The HTTP protocol is stateless because once it will end, it will get terminated completely. It does not save any information for particular session activity. So, the information is saved and later all the information is gathered from the cookies because cookies are nothing but the one which saves the user information.
Sessions in Codeigniter with examples
All of the information saved on the client-side and sessions is the one that maintains the interaction to the server-side. This is a basic difference between both of them. One more thing is cookies store information in the end-user browser until the user deletes it or its time doesn’t get over. The session is a server-side data stored on a temporary basis. As the user closes the browsers, the session will automatically get removed. That is a basic difference between both of them.
- Show more and show less content using jQuery
- Best PHP IDEs And Top PHP Editors And Development Tools
- How To Convert String To Array In PHP By PHP Explode() Function
When we understand from PHP concept for any PHP framework, here for CodeIgniter, we should keep this picture in our mind. We are going to use cookies as well as sessions while developing a web application so we got a brief description or a basic difference between both of them. In this picture, you can also see that I am taking some parameters over here with the cookie, session ID and the content as ‘Suzan’. Once the access is verified from the server-end then, the server will send the data to the cookies, to store in them. And cookie saves the information into its system and it keeps this information with respect to the identification of a user. And the server will identify the user indirectly. That is the way interaction takes place from both of them.
Session management in CodeIgniter
While building websites we often need to track the user’s activity and we have to use the session for that purpose so we will understand the session management with CodeIgniter. CodeIgniter has a session class for this purpose, which manages everything related to the user’s activity with the stipulated time period. Let’s have a look at initializing a session.
Initializing a session in CodeIgniter
First of all, we understand how the session management works with CodeIgniter. Initializing a session is completely a direct order this load with the library, the session library.
This can be any kind of session that CodeIgniter needs to start. After loading a session, you need to add data that is not meant ordered in this session. After that, we have to add session data. Adding a session data means some variables to get associated with them. This will be in PHP, so we simply use $_SESSION to set the data and same format is followed over here. We have a session and inside that, we have the key and the associated value for it. Adding a session data in CodeIgniter is easily done with the set_userdata() function of the session library. This data can be any key, value, or a number of arrays and the associative arrays with the values that can be possible. It’s up to you and based on the requirement that what should be displayed on the screen.
set_userdata() function to set session in CodeIgniter
set_userdata() function takes the arguments in two ways. You can either pass two arguments in which the first one will be the key and the second one will be the value. Another way is to pass an array which itself is a key, value pair. The first method is useful in the case when you want to set a single session value to a single key. The value, in this case, can also be an array. That will be stored in the session key itself. However, if you want to store multiple session value at one go, you can pass them in array form. Let’s take a look at the example below.
Example of set_userdata()
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
#Setting values one by one $this->session->set_userdata('username', 'techbriefers'); $this->session->set_userdata('email', 'techbriefers @gmail.com'); $this->session->set_userdata('logged_in', true); $this->session->set_userdata('permissions', array('manageUsers', 'manageProducts')); #Setting multiple values in one go $my_sess_data = array( 'username' => 'techbriefers', 'email' => 'techbriefers @gmail.com', 'logged_in' => true, 'permissions' => array('manageUsers', 'manageProducts') ); $this->session->set_userdata($my_sess_data); |
unset_userdata() function to remove session in CodeIgniter
Now comes: the process to remove session data. Removing a session data means like in PHP we can remove the data using unset functions. Similarly removing a session data in CodeIgniter is very simple it is just with the simple function call that is unset_userdata() with the values.
Example of removing a single session data:
1 2 |
$this->session->unset_userdata('username'); $this->session->unset_userdata('email'); |
Session name is nothing but the key-value pair. So automatically the value for that key associated with it will be removed. If you want to remove more than one value, we can also do that by, passing the session array name instead of giving each key separately to the function parameter. We just need to create an array to set key names to be removed or unset and pass that array as a function argument, like in the example below.
Example of remove multiple session data at one go:
1 2 |
$my_sess_unset= array('logged_in', 'permissions'); $this->session->unset_userdata($my_sess_unset); |
Now we have to fetch the data stored in the session. We can do this by using userdata() function of the session library. After setting up the data we can retrieve that data by this function. I will proceed with the data in the example above. As I set the values for keys: username, email, etc. Then the example below will lead you to retrieve the session data stored on the particular keys. This function takes one argument, the key name, on which the value is assigned. If there is no data assigned on the key passed as an argument, the function will return a null value.
Example to fetch or retrieve session values is:
1 2 3 4 |
echo $this->session->userdata('username') . '<br>'; echo $this->session->userdata('email') . '<br>'; echo $this->session->userdata('logged_in') . '<br>'; print_r($this->session->userdata('permissions')); |
Let’s create a simple app where the user will enter the name which will be set in the session and show on a page. On the same page, we will create a link to the action where that data will get unset. So let’s move towards the codebase.
The first thing I am doing is creating a controller with ‘Mysession‘ name. Inside the index method of this controller, I will make the code to show the view containing a form. This form will contain a field to input name and a button to submit that value.
After this, I am creating add() method, to which form will be submitted. The variable $uname will store stored the form value at key ‘uname’, that we will assign to the session key ‘username’. This action/method will show a view ‘sess-view’, which shows the session name that we are going to set from the controller, in a heading. Below the name text, I am creating a link that will take the user to remove action.
In remove() action of this controller, there is a simple code to unset the session key ‘username’. After unsetting the session data, the action will redirect the user to the index action of this controller.
As you know, before using any library in CodeIgniter, we need to load that. I am loading the session library in the constructor of this controller. Loading a library in the constructor will make it available throughout all the actions. You can also load it in each action separately.
Code to demonstrate set and unset session in CodeIgniter:
Example code of form view (sess-form.php)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 |
<!DOCTYPE html> <html> <head> <title>Codeigniter Session Demo | techbriefers.com</title> </head> <body> <div style="text-align: center" > <form action="<?php echo base_url('mysession/add') ?>" method="post"> <input type="text" placeholder="Enter Name" name="uname"> <button type="submit">Set Name</button> </form> </div> </body> </html> Example code of view showing session name (sess-view.php) <!DOCTYPE html> <html> <head> <title>Codeigniter Session Demo | techbriefers.com</title> </head> <body> <div style="text-align: center" > <h1>Welcome <?php echo $this->session->userdata('username'); ?></h1> <a href="<?php echo base_url('mysession/remove'); ?>">Remove Session</a> </div> </body> </html> |
Example code of Controller (Mysession.php)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 |
<?php defined('BASEPATH') OR exit('No direct script access allowed'); class MySession extends CI_Controller { public function __construct() { parent::__construct(); $this->load->library('session'); } public function index() { $this->load->view('sess-form'); } public function add() { $uname = $this->input->post('uname'); $this->session->set_userdata('username', $uname); $this->load->view('sess-view'); } public function remove() { $this->session->unset_userdata('username'); redirect(base_url('mysession')); } } |
Hope this article will help you to handle and understand sessions in CodeIgniter. You can have a look at these articles too.
This is an informative post. Got a lot of info and details from here. Thank you for sharing this and looking forward to reading more of your post.
very bad