How to read all files and directories in a folder | PHP glob()
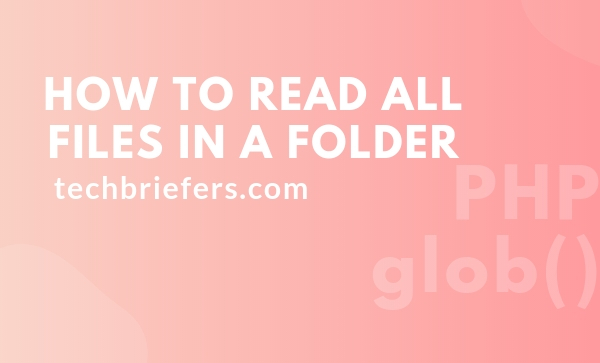
To read all the files in a particular directory using PHP, we can use glob() function. This function allows to read any (pathname) file/ sub directory/ both in a particular directory (in which files are being searched).
Definition:
The glob() function returns an array of filenames or directories matching a specified pattern(given pattern as first argument). The glob() function returns an array containing the matched files/directories names, an empty array if no file/directory is matched and FALSE if any error occurs.
Syntax of glob() function (as on official website)
1 |
glob ( string $pattern [, int $flags = 0 ] ) : array |
Description
glob() function uses two parameters. First is mandatory string pattern and second is optional integer (flag).
How to use glob() function
First parameter is a string which is treated as pattern to search pathnames (files/directories).
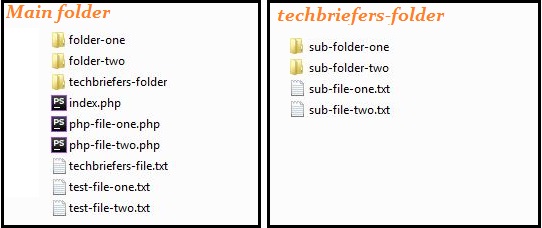
Examples of glob() function by patterns
Eg-1: To search any file or directory
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 |
$search_results = glob('*'); print_r($search_results); /*output Array ( [0] => folder-one [1] => folder-two [2] => index.php [3] => php-file-one.php [4] => php-file-two.php [5] => techbriefers-file.txt [6] => techbriefers-folder [7] => test-file-one.txt [8] => test-file-two.txt ) */ |
Eg-2: To search only PHP files
1 2 3 4 5 6 7 8 9 10 11 |
$search_results = glob('*.php'); print_r($search_results); /*output Array ( [0] => index.php [1] => php-file-one.php [2] => php-file-two.php ) */ |
Eg-3: To search only text files
1 2 3 4 5 6 7 8 9 10 11 |
$search_results = glob('*.txt'); print_r($search_results); /*output Array ( [0] => techbriefers-file.txt [1] => test-file-one.txt [2] => test-file-two.txt ) */ |
Eg-4: To search any file or directory starts with ‘a’
1 2 3 4 5 6 7 8 |
$search_results = glob('a*'); print_r($search_results); /*output Array ( ) */ |
Eg-4: To search any file or directory starts with ‘tech’
1 2 3 4 5 6 7 8 9 10 |
$search_results = glob('tech*'); print_r($search_results); /*output Array ( [0] => techbriefers-file.txt [1] => techbriefers-folder ) */ |
Eg-5: To search any file or directory contains ‘brie’
1 2 3 4 5 6 7 8 9 10 |
$search_results = glob('*brie*'); print_r($search_results); /*output Array ( [0] => techbriefers-file.txt [1] => techbriefers-folder ) */ |
Eg-6: To search any file or directory that starts with ‘tech’ and end with ‘folder’
1 2 3 4 5 6 7 8 9 |
$search_results = glob('tech*folder'); print_r($search_results); /*output Array ( [1] => techbriefers-folder ) */ |
Second parameter is an integer which should be a valid flag.
Valid flags:
GLOB_MARK – Adds a slash to the end each directory name found
GLOB_NOSORT – Return files as they appear in the directory without sorting alphabetically. When this flag is not used, the pathnames are sorted alphabetically
GLOB_NOCHECK – Return the search pattern if no files matching it were found
GLOB_NOESCAPE – Backslashes do not quote metacharacters
GLOB_BRACE – Expands {a,b,c} to match ‘a’, ‘b’, or ‘c’
GLOB_ONLYDIR – Return only directory entries which match the pattern
GLOB_ERR – Stop on read errors (like unreadable directories), by default errors are ignored.
Examples of glob() function by flags
Eg-7: To add slash at the end of directory
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 |
$search_results = glob('*', GLOB_MARK); print_r($search_results); /*output Array ( [0] => folder-one/ [1] => folder-two/ [2] => index.php [3] => php-file-one.php [4] => php-file-two.php [5] => techbriefers-file.txt [6] => techbriefers-folder/ [7] => test-file-one.txt [8] => test-file-two.txt ) */ |
Eg-8: To see unsorted list of files and directories
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 |
$search_results = glob('*', GLOB_NOSORT); print_r($search_results); /*output Array ( [0] => test-file-one.txt [1] => index.php [2] => techbriefers-file.txt [3] => php-file-one.php [4] => php-file-two.php [5] => test-file-two.txt [6] => folder-two [7] => folder-one [8] => techbriefers-folder ) */ |
Eg-9: If file/folder is not found returns pattern, without using GLOB_NOCHECK returns an empty array
1 2 3 4 5 6 7 8 9 |
$search_results = glob('new*', GLOB_NOCHECK); print_r($search_results); /*output Array ( [0] => new* ) */ |
Clear difference can bee seen if you compare Eg-9 and Eg-4. In Eg-4 results are not found hence empty array is returned.
Eg-10: To search only directories (other files are ignored in search)
1 2 3 4 5 6 7 8 9 10 11 |
$search_results = glob('*', GLOB_ONLYDIR); print_r($search_results); /*output Array ( [0] => folder-one [1] => folder-two [2] => techbriefers-folder ) */ |
Searching/ pathnames (files and folders in a paricular sub folder)
Eg-11: To search files and folders in ‘techbriefers-folder’ directory
1 2 3 4 5 6 7 8 9 10 11 12 |
$search_results = glob('techbriefers-folder/*'); print_r($search_results); /*output Array ( [0] => techbriefers-folder/sub-file-one.txt [1] => techbriefers-folder/sub-file-two.txt [2] => techbriefers-folder/sub-folder-one [3] => techbriefers-folder/sub-folder-two ) */ |
***I have used echo ‘<pre>’; before printing array to show in a user friendly view.
The best I’ve seen so far. Thumbs up for a very good and easy to understand page.