How to check variable types in PHP
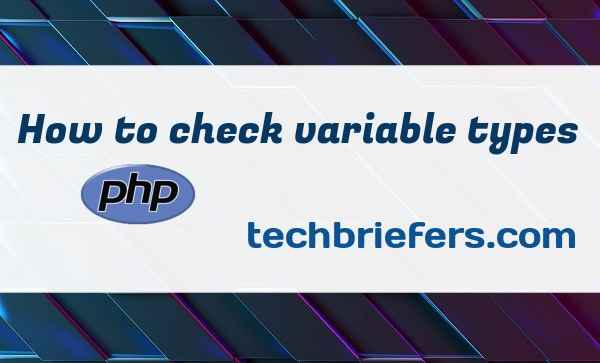
To check variable types in PHP, we have many variable handling functions available. Below is the list of functions I am going to explain.
- Click to read about PHP variables.
- Click to read about data types.
- Click to read about how to check data types.
Function list to check variable types.
- is_array()
- is_bool()
- is_double()
- is_float()
- is_int()
- is_long()
- is_integer()
- is_numeric()
- is_object()
- is_resource()
- is_null()
- is_string()
is_array() function in PHP
PHP is_array() function checks whether the passed argument is an array type or not.
Returns true if argument is array, otherwise returns false.
$arr = [1, 2];
var_dump(is_array($arr));
/*
result is
bool(true)
*/
$arr = 'not array';
var_dump(is_array($arr));
/*
result is
bool(false)
*/
is_bool() function in PHP
PHP is_bool() function checks whether the passed argument is of Boolean type (true/false) or not.
Returns true if argument is Boolean type, otherwise returns false.
$var = 1;
var_dump(is_bool($var));
/*
result is
bool(true)
*/
$var = true;
var_dump(is_bool($var));
/*
result is
bool(false)
*/
is_double() function in PHP
PHP is_double() function checks whether the passed argument is of double/float/real type or not.
Returns true if argument is double/float/real type, otherwise returns false.
is_float() function in PHP
PHP is_float() function is alias of is_double() function.
is_real() function in PHP
PHP is_real() function is alias of is_double() function.
$var = 1.3;
var_dump(is_double($var));
var_dump(is_float($var));
var_dump(is_real($var));
/*
result is
bool(true)
bool(true)
bool(true)
*/
$var = 02;
var_dump(is_double($var));
var_dump(is_float($var));
var_dump(is_real($var));
/*
result is
bool(false)
bool(false)
bool(false)
*/
is_int() function in PHP
PHP is_int() function checks whether the passed argument is is of type integer or not.
Returns true if argument is integer type, otherwise returns false.
is_long() function in PHP
PHP is_long() function is alias of is_int() function.
is_integer() function in PHP
PHP is_integer() function is alias of is_int() function.
$var = 100;
var_dump(is_int($var));
var_dump(is_long($var));
var_dump(is_integer($var));
/*
result is
bool(true)
bool(true)
bool(true)
*/
$var = 10.0;
var_dump(is_int($var));
var_dump(is_long($var));
var_dump(is_integer($var));
/*
result is
bool(false)
bool(false)
bool(false)
*/
is_numeric() function in PHP
PHP is_numeric() function checks whether the passed argument is a numeric type value or not.
Returns true if argument is numeric type, otherwise returns false.
$var = 100;
var_dump(is_numeric($var));
/*
result is
bool(true)
*/
$var = '100';
var_dump(is_numeric($var));
/*
result is
bool(true)
*/
$var = 'hello';
var_dump(is_numeric($var));
/*
result is
bool(false)
*/
is_null() function in PHP
PHP is_null() function checks whether the passed argument is a null value or not.
Returns true if argument is null, otherwise returns false.
$var = 100;
var_dump(is_null($var));
/*
result is
bool(false)
*/
$var = '';
var_dump(is_null($var));
/*
result is
bool(false)
*/
$var = null;
var_dump(is_null($var));
/*
result is
bool(true)
*/
is_object() function in PHP
PHP is_object() function checks whether the passed argument is an object i.e, instance of class or not.
Returns true if argument is instance of class, otherwise returns false.
class MyClass {
}
$obj = new MyClass();
var_dump(is_object($obj));
/*
result is
bool(true)
*/
is_resource() function in PHP
PHP is_resource() function checks whether the passed argument is a resource or not.
Returns true if argument is a resource, otherwise returns false.
$value = fopen(__DIR__.'/text.txt','r');
var_dump(is_resource($value));
fclose($value);
is_string() function in PHP
PHP is_string() function checks whether the passed argument is a string or not.
Returns true if argument is a string, otherwise returns false.
$var = "mystring";
var_dump(is_string($var));
/*
result is
bool(true)
*/
$var = "101";
var_dump(is_string($var));
/*
result is
bool(true)
*/
$var = 101;
var_dump(is_string($var));
/*
result is
bool(false)
*/
Leave a Reply