Operators in PHP
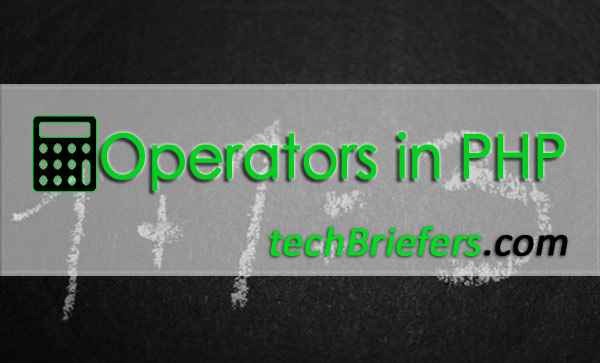
While trying to learn about PHP operators, the first question is What is operator?
PHP Operators are symbols which operate one or more operands, just like in mathematical expressions.
PHP Operators
PHP operators can be grouped in the following categories:
- Arithmetic Operators.
- Assignment Operators.
- Comparison Operators.
- Incrementing/Decrementing Operators.
- Logical Operators.
- String Operators.
- Array Operators.
PHP Arithmetic Operators
These operators are used to do arithmetic operations i.e. addition, substraction etc on operands (variables). The explanation would be easier with the table below.
Operator | Name | Expression | Result |
+ | Addition | $x + $y | Sum of $x and $y |
– | Subtraction | $x – $y | Difference of $x and $y |
* | Multiplication | $x * $y | Product of $x and $y |
/ | Division | $x / $y | Quotient of $x and $y |
% | Modulus | $x % $y | Remainder of $x divided by $y |
** | Exponentiation | $x ** $y | Result of raising $x to the $y’th power (Introduced in PHP 5.6) |
PHP Assignment Operators
These operators are used to write a value to a variable. The explanation would be easier with the table below. The basic assignment operator is “=”.
The value of an assignment expression is the final value assigned to the variable. In addition to the regular assignment operator “=”, some other assignment operators are combinations of an operator followed by an equal “=” sign.
- +=
- -=
- *=
- /=
- %=
The explanation would be easier with the table below.
Expression | Shorthand | Description |
$a = $a + $b | $a+= $b | Adds 2 numbers and assigns the result to the first. |
$a = $a -$b | $a-= $b | Subtracts 2 numbers and assigns the result to the first. |
$a = $a*$b | $a*= $b | Multiplies 2 numbers and assigns the result to the first. |
$a = $a/$b | $a/= $b | Divides 2 numbers and assigns the result to the first. |
$a = $a%$b | $a%= $b | Computes the modulus of 2 numbers and assigns the result to the first. |
PHP Comparison Operators
Comparison operators allow to compare two values.
If you compare a number with a string or the comparison involves numerical strings, then each string is automatically converted to a number and the comparison performed numerically. These rules also apply to the switch statement. The type conversion does not take place when the comparison is === or !== as this involves comparing the type as well as the value.
Example | Name | Result |
$a == $b | Equal | TRUE if $a is equal to $b after type juggling |
$a === $b | Identical | TRUE if $a is equal to $b, and they are of the same type. |
$a != $b | Not equal | TRUE if $a is not equal to $b after type juggling. |
$a <> $b | Not equal | TRUE if $a is not equal to $b after type juggling. |
$a !== $b | Not identical | TRUE if $a is not equal to $b, or they are not of the same type. |
$a < $b | Less than | TRUE if $a is strictly less than $b. |
$a > $b | Greater than | TRUE if $a is strictly greater than $b. |
$a <= $b | Less than or equal to | TRUE if $a is less than or equal to $b. |
$a >= $b | Greater than or equal to | TRUE if $a is greater than or equal to $b. |
$a <=> $b | Spaceship | An integer less than, equal to, or greater than zero when $a is respectively less than, equal to, or greater than $b. Available as of PHP 7. |
PHP Incrementing & Decrementing Operators
Increment and decrement operators are unary operators i.e. need only one operand that add or subtract one from their operand, respectively.
Result
Example | Name |
++$a | Pre-increment |
$a++ | Post-increment |
–$a | Pre-decrement |
$a– | Post-decrement |
PHP Logical Operators
An expression that has logical operator returns either 0 or 1 depending upon whether expression results true or false. Logical operators are generally used in decision making.
Result
Example | Name |
$a and $b | And |
$a or $b | Or |
$a xor $b | Xor |
! $a | Not |
PHP String Operators
String operators are of two types. The first is the concatenation operator “.”, which returns the concatenation of its right and left value(s). The second is the concatenating assignment operator “.=”, which appends the value on the right side to the value on the left side.
<?php $a = "Hello"; $b = "World"; echo $a . " " . $b; /* Result will be * Hello World */ $a .= " World"; // can be used as $a .= "".$b; echo $a; /* Result will be * Hello World */ ?>
PHP Array Operators
Operations on arrays are performed using Arrays Operators like Union, Equality, Identity, Inequality and Non-identity. The explanation would be easier with the table below
Result
Example | Name |
$x + $y | Union |
$x == $y | Equality |
$x === $y | Identity |
$x != $y | Inequality |
Read explained PHP Array Operators
Leave a Reply